I saw a post on Bluesky that proposed a problem that I had been thinking about for a long time!
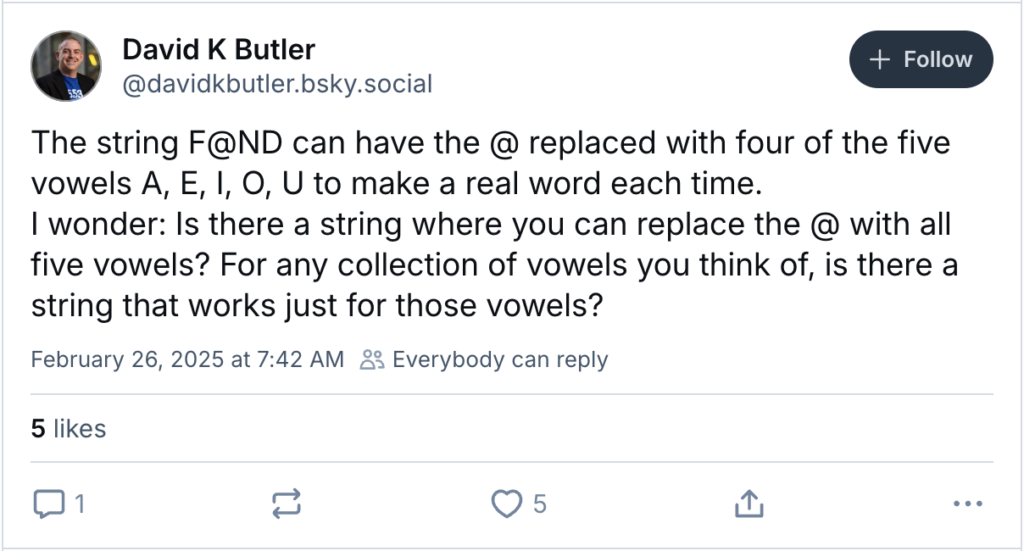
I figured that with the help of dictionary and a Python script, I could find the desired examples.
One vowel
- A: t_lk
- E: wh_n
- I: w_th
- O: fr_m
- U: cl_b
Two vowels
- AE: sm_ll
- AI: th_nks
- AO: d_wn
- AU: b_nk
- EI: m_nd
- EO: kn_w
- EU: j_st
- IO: sh_rt
- IU: sk_lls
- OU: sp_rt
Three vowels
- AEI: w_ll
- AEO: l_ss
- AEU: fl_sh
- AIO: t_p
- AIU: st_ff
- AOU: c_ts
- EIO: m_ld
- EIU: d_sk
- EOU: c_lts
- IOU: p_lls
Four vowels
- AEIO: r_d
- AEIU: b_ll
- AEOU: l_gs
- AIOU: m_st
- EIOU: d_ll
- AEIOU: b_t
Python code
Here’s my quick Python script to help find these. The dictionary has some words that I’m not familiar with (e.g. “cen,” “wir,” etc.) so I had to manually audit the results.
from wordfreq import top_n_list
import re
from collections import defaultdict
common_words = top_n_list('en', 50000)
def vowels(word):
return re.findall(r'[aeiouy]', word, re.IGNORECASE)
def has_one_vowel(word):
return len(vowels(word)) == 1
def first_vowel(word):
return vowels(word)[0]
def replace_vowels(word):
return re.sub(r'[aeiouyAEIOUY]', '_', word)
one_vowel_words = filter(has_one_vowel, common_words)
dict = defaultdict(list)
for w in one_vowel_words:
dict[replace_vowels(w)] = sorted(dict[replace_vowels(w)] + [first_vowel(w)])
vowel_subsets = [['a'], ['e'], ['i'], ['o'], ['u'], ['a', 'e'], ['a', 'i'], ['a', 'o'], ['a', 'u'], ['e', 'i'], ['e', 'o'], ['e', 'u'], ['i', 'o'], ['i', 'u'], ['o', 'u'], ['a', 'e', 'i'], ['a', 'e', 'o'], ['a', 'e', 'u'], ['a', 'i', 'o'], ['a', 'i', 'u'], ['a', 'o', 'u'], ['e', 'i', 'o'], ['e', 'i', 'u'], ['e', 'o', 'u'], ['i', 'o', 'u'], ['a', 'e', 'i', 'o'], ['a', 'e', 'i', 'u'], ['a', 'e', 'o', 'u'], ['a', 'i', 'o', 'u'], ['e', 'i', 'o', 'u'], ['a', 'e', 'i', 'o', 'u']]
for vs in vowel_subsets:
print("".join(vs).upper() + ":", ", ".join([k for k, v in dict.items() if v == vs][0:10]))
Leave a Reply